Flutter is a powerful platform for developing mobile applications, but it also provides the tools to create and distribute packages. A Flutter package is a reusable collection of code that can be easily shared and integrated into other projects. In this article, we’ll go through the process of creating a simple counter package for Flutter.
Step 1: Creating a New Flutter Package
To create a new Flutter package, open your preferred code editor (such as Android Studio or Visual Studio Code) and select “Create New Flutter Project”. Choose “Flutter Package” as the project type and give your package a name.
This will create a new directory for your package with the following files:
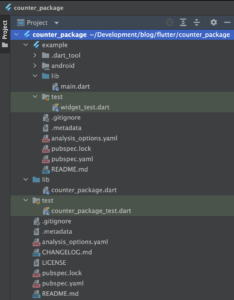
Folder Structure
Step 2: Creating the Counter Class
In the lib/
directory, create a new file called counter.dart
. This file will contain the code for our Counter
class.
class Counter {
int _count = 0;
int get count => _count;
void increment() {
_count++;
}
void decrement() {
_count--;
}
}
In this code, we define a Counter
class that has an _count
variable and two methods: increment()
and decrement()
. The increment()
method increases the count by 1 and the decrement()
method decreases the count by 1. The count
property returns the current count value.
Step 3: Specifying Package Information in pubspec.yaml
The pubspec.yaml
file is used to specify information about your package, such as its name, version, and dependencies. Open the pubspec.yaml
file and add the following information:
name: counter_package
description: A new Flutter project.
version: 0.0.1
environment:
sdk: '>=2.18.1 <3.0.0'
flutter: ">=1.17.0"
dependencies:
flutter:
sdk: flutter
dev_dependencies:
flutter_test:
sdk: flutter
flutter_lints: ^2.0.0
flutter:
In this code, we set the name
, description
, and version
of our package. We also specify that our package depends on the Flutter SDK.
Step 4: Publishing Your Package
Before publishing your package, make sure to run the following command in the root directory of your package to ensure that all dependencies are up to date:
flutter packages get
To publish your package on pub.dev, you’ll need to create an account and an API token. Once you have these, run the following command:
flutter pub publish
This will upload your package to pub.dev and make it available for other developers to use.
Step 5: Using Your Package
To use your package in another Flutter project, add it as a dependency in the pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
counter_package: ^1.0.0
Then, import the package in your code:
import 'package:counter_package/counter.dart';
final counter = Counter();
counter.increment();
print(counter.count); // prints 1
Conclusion
In this article, we’ve covered the process of creating a simple counter package for Flutter. We’ve discussed creating a new Flutter package, defining a Counter
class, specifying package information in the pubspec.yaml
file, publishing the package on pub.dev, and using the package in another Flutter project.
By following these steps, you can create your own packages
This is a fantastic resource for anyone who wants to create their own Flutter package. The guide is easy to follow, and the author clearly knows what they’re talking about.
This step-by-step guide on creating a Flutter package is a lifesaver! As an aspiring Flutter developer, I’ve been eager to contribute to the Flutter community by building and sharing my own packages. Your detailed explanations and code examples have made the process much more approachable. Thank you for empowering us to create and distribute our Flutter packages!
I’ve always wondered how to create a Flutter package, and your article provided the perfect answer! Your clear and concise instructions, along with the best practices you shared, have given me the confidence to start building my own packages. The tips on versioning, documentation, and publishing are invaluable. Thank you for sharing your expertise!
Jennifer, I completely agree with you! This step-by-step guide has been a game-changer for me. It demystified the process of creating a Flutter package and gave me the knowledge I needed to contribute to the Flutter ecosystem. The explanations are thorough, and the accompanying examples make it easy to follow along. Thanks for sharing your insights!
This step-by-step guide for creating a Flutter package was exactly what I needed. The instructions were clear, and the additional tips and best practices were invaluable. I successfully published my first package following this guide. Highly recommended for Flutter developers!